Let’s Talk About the Changing Face of Test Automation ⚛️
Testing automation is evolving faster than ever. There’s a shift in focus—from UI testing to API, unit, and contract testing. This transformation largely stems from the rise of microservices, containerization, and APIs. While UI automation remains essential, it often lags in identifying defects caused by backend issues. As testing shifts towards API layers, understanding the “why” and “how” of API testing can make a tremendous impact on software quality and the speed of test execution.
As Michael Bolton famously said, “Testing is not the art of finding bugs. It’s the art of finding useful information about quality.”
Visualization: API vs. UI Testing Mind Maps 🧠
To visualize the differences, here’s a mind map comparing API and UI testing focuses:
API testing directly tests the backend business logic and skips potential flakiness seen in UI tests, leading to fewer brittle tests.
Why the Shift to API Testing? 🛠️
APIs power much of today’s software functionality, especially in distributed systems and microservice-based architectures. Testing the UI alone often doesn’t reveal issues in the API interactions between microservices, leading to hidden issues in backend operations.
Benefits of Focusing on API Testing 🖥️
- Early Defect Detection: API tests can be executed early in the development lifecycle, identifying defects before they impact other components.
- Faster Feedback Loops: Since API tests are generally quicker to run and simpler to maintain than UI tests, they facilitate rapid feedback.
- Stability Across Platforms: APIs are usually less volatile than UIs, making tests more stable and maintainable.
Types of API Testing
Here’s a quick breakdown of the core types of API testing and their purposes:
Type | Purpose |
---|---|
Functional | Validates API responses for expected outputs |
Security | Assesses API’s security by validating authentication, authorization, etc. |
Load | Examines performance under heavy load conditions |
Contract | Verifies consistency in API request/response format |
Integration | Ensures correct interaction between different API components |
Each type targets different aspects of API functionality, security, and robustness, forming a well-rounded API testing approach.
API Testing Tools and Techniques 🛠️
Let’s look at some popular tools and techniques used for API testing.
- Postman: Widely used for manual testing, it allows quick API requests and responses with scripting support for automation.
- REST Assured: A Java library that provides comprehensive support for automating REST APIs.
- SoapUI: Popular for testing SOAP and REST services with an intuitive interface.
- Swagger: Helpful for contract testing, it ensures consistent API documentation and contract compliance.
- WireMock: A simulation tool to test APIs by creating stubs and mocks, useful in testing without actual backend dependencies.
Practical Bottlenecks in API Testing and Solutions
- Managing Test Data in APIs: APIs often require specific data formats, and mismatches can lead to failures.
- Solution: Use a centralized test data management solution or test data injection techniques to standardize input and output data.
- API Rate Limits: Many APIs limit the number of requests per second, leading to throttling issues.
- Solution: Utilize tools like JMeter for load testing to identify rate limit thresholds. Alternatively, work with API gateways like Kong that handle rate limiting gracefully.
Example: A Practical Guide to API Testing 🔍
To help clarify, let’s look at a simple example of API testing with Postman for a fictional e-commerce platform.
Test Setup:
API Endpoint: https://api.shopxyz.com/v1/products
Method: GET
Headers: Content-Type: application/json
Expected Output: List of product details in JSON format.
Testing Process:
Open Postman and enter the endpoint URL.
Set the method to GET, add headers, and click “Send”.
Validate the response for a 200 Status Code, ensuring the response contains valid product details.
Advanced Validation:
Create a test script within Postman to check if the response format matches the expected schema, using a JSON schema validator.
The Role of Contract Testing in API Reliability
Contract testing ensures that any change in API structure or format is flagged before it affects dependent services. For example, if a field is removed or renamed, contract tests will notify developers immediately, reducing the risk of breaking integrations.
“API contract testing reduces friction between frontend and backend teams, allowing changes to be detected and resolved early on,” says a leading API architect.
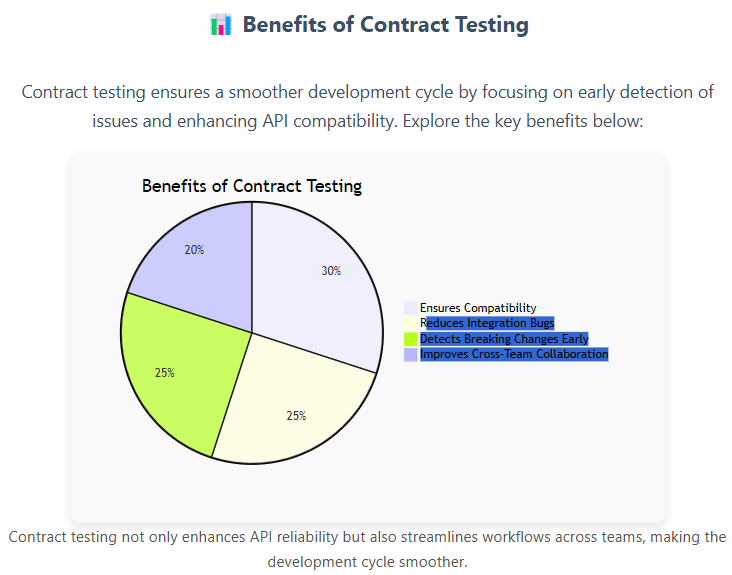
Step-By-Step Guide for a Basic API Test Automation Framework (Java + REST Assured) 💻
javaCopy codeimport io.restassured.RestAssured;
import io.restassured.response.Response;
import static io.restassured.RestAssured.*;
import static org.hamcrest.Matchers.*;
public class APITest {
public static void main(String[] args) {
RestAssured.baseURI = "https://api.shopxyz.com/v1";
Response res = given()
.header("Content-Type", "application/json")
.when()
.get("/products")
.then()
.statusCode(200)
.body("products.size()", greaterThan(0))
.extract()
.response();
System.out.println("Response: " + res.asString());
}
}
This framework is perfect for small, repeatable tests and can be integrated with CI/CD tools like Jenkins to maintain continuous testing flow.
Common Pitfalls in API Testing 🕳️
Flaky Tests Due to Unstable Environments:
- If the environment is frequently changing, API responses can vary.
- Solution: Use mock servers like WireMock for testing without relying on the live environment.
Improper Authorization Handling: - APIs often require specific token-based authorizations, which can lead to failures if not managed correctly.
- Solution: Use a token management solution to handle different authorization schemes.
Wrapping Up: The Frontline of Testing 🏁
API testing offers a significant advantage by enabling early-stage defect detection, improving test speed, and reducing dependency on the UI. For testers transitioning to API-based testing, investing time in learning these techniques can greatly enhance their testing toolbox. For more details, check out related articles on contract testing here and API test automation here.
By adopting a shift-left approach with API testing, we’re not just following a trend but building a more robust, adaptable testing framework suited for the modern development landscape.